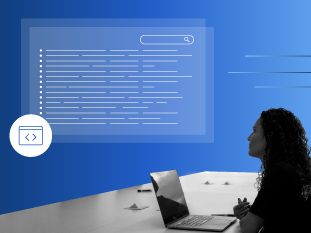
Good Naming Practices in Software Development
In our everyday lives, people often assign names to various things, such as other people, pets, vehicles, and more. These names are typically chosen based on personal preferences, geographical location, cultural heritage, trends, or simply because they sound amusing.
Let's consider some examples:
- A father might name his child after himself as an expression of pride and a desire to continue a family tradition. Alternatively, if he is an avid sports fan, he might name the child "Cristiano Ronaldo" due to the popularity of the soccer player at that time.
- When a new residential complex is being built, and one or more almond trees are on the land, the complex might be named "The Almond."
- A family might adopt a pet and decide to give it a humorous or unusual name, such as "Bacon," "Chewbacca," or "Cupcake."
While these choices are entirely valid in everyday life and reflect individual tastes and preferences, in the context of software development, we must adopt a more cautious approach. In this blog post, we’ll explore the importance of proper naming in software development, including code examples, best practices, and naming conventions.
Naming Practices in Software Development
Software developers constantly create and assign names to various elements, including projects, modules, classes, variables, methods, databases, tables, and columns. In this environment, choosing appropriate names is crucial because it impacts the readability, maintainability, and understanding of the software we create. Therefore, it is essential to embrace consistent and meaningful naming practices in software development.
What Does Good Naming Mean in Software Development?
According to Robert C. Martin’s book Clean Code, choosing a good name takes time but saves more time in the long run. The name of a variable, function, or class should address all significant questions; it should reveal why it exists, what its purpose is, and how it's utilized. If a name needs a comment, then it doesn't effectively reveal its intent.
Choosing names that reveal intent makes it much easier to understand and modify code. For example, consider this piece of code from Clean Code:
public List<int[]> getThem() {
List<int[]> list1 = new ArrayList<int[]>();
for (int[] x : theList) {
if (x[0] == 4) list1.add(x);
}
return list1;
}
What makes it challenging to discern the purpose of this code? There are no complex expressions, and the spacing and indentation appear reasonable. The code references only three variables and two constants, without any elaborate classes or polymorphic methods; it seems to consist solely of an array list. The issue here lies not in the code's simplicity but in its implicit nature. The context is not explicitly conveyed within the code itself.
Why is Proper Nomenclature Important in Software Development?
Following proper naming practices is essential to writing clean and maintainable code. Failing to do so can result in the following issues:
- Lack of clarity: Poorly named variables, functions, classes, or other code elements make it difficult to understand the code. If developers cannot easily determine the purpose or functionality of a component from its name, working with the code becomes challenging.
- Misinterpretation: Ambiguous or misleading names can cause developers to misunderstand how a particular element works, potentially leading to bugs or incorrect behavior in the software.
- Maintenance challenges: Poorly named code can be hard to maintain. When you or other developers revisit the code for updates or bug fixes, unclear or inconsistent names can slow down the process, increasing the likelihood of introducing new issues.
- Communication breakdowns: Software development often involves team collaboration. Poor naming can hinder effective communication, as team members may need extra time to decipher code or ask for clarification.
- Scalability issues: As software projects grow in size and complexity, proper naming becomes even more critical. Bad naming practices can accumulate over time, making scaling and extending the software increasingly difficult.
- Maintainability costs: Fixing poor naming after the fact can be costly and time-consuming. This often involves refactoring code and updating documentation, which can affect other parts of the software.
Here’s an example of code that uses bad variable names from the book Code Complete, 2nd Edition by Steve McConnell:
Java Example of Poor Variable Names
x = x - xx;
xxx = fido + SalesTax( fido ); x = x + LateFee( x1, x ) + xxx; x = x + Interest( x1, x );
Now, let's look at an improved version of the same code that enhances readability and simplifies understanding, also from Code Complete, 2nd Edition:
Java Example of Good Variable Names
balance = balance - lastPayment;
monthlyTotal = newPurchases + SalesTax( newPurchases );
balance = balance + LateFee( customerID, balance ) + monthlyTotal; balance = balance + Interest( customerID, balance );
In the second example, you realize the benefits of taking the time to give meaningful names to variables.
Basic Principles of Creating Meaningful Names
Principle | Description |
Use intention-revealing names | The name of the variable, method, or class should explain why it exists, what it does, and how it’s used. |
Avoid disinformation | Avoid poor or lazy naming. For example: var ro, looks like a good repair order, but it could be misinformative. |
Make meaningful distinctions | Be careful not to use similar variable names in the same context. Ie. using RepairOrderInfo and RepairOrderData in the same RepairOrder object. A clear distinction must be made. |
Use pronounceable names | Choose names that are easy to pronounce and understand when spoken.
|
Use searchable names | Choose names for variables, functions, classes, and other code elements that are easy to search for within the codebase.
|
Member prefixes | Prefixes provide clarity and indicate the scope of a variable, making it easier to understand its context.
|
Class names | Classes and objects should have noun or noun phrase names like “Customer”; a class name should not be a verb.
|
Method names | Methods should have verb or verb phrase names like “postPayment”, “deletePage”, or “save”. |
Maintain language consistency | To maintain code clarity, avoid mixing languages when naming variables. Ensure that your variable names are consistently in the same language throughout the codebase (ie. all in English, or all in Spanish).
|
Avoid being overly creative | Cuteness in code can manifest through colloquialisms, slang, or overly clever names. For instance, refrain from giving a method an attention-grabbing name like "GodsHand(id)" for a simple deletion function.
|
Software Naming Conventions
Remember that naming conventions can vary between programming languages and communities, so it's important to adapt to the specific guidelines relevant to your project or organization. Let's examine some commonly used delimiting conventions in code:
Convention Name | Description | Example |
Snakecase | Words are delimited by an underscore. | first_name
|
Pascalcase | Words are delimited by capital letters. | FirstName
|
Camelcase | Words are delimited by capital letters, except the initial word. | firstName
|
When naming variables, two fundamental principles to keep in mind are consistency and readability. It's crucial to maintain a consistent approach to naming conventions across your codebase. Consistency enhances your code's readability and ensures that others can better understand it.
The Anecdote
Utilizing proper naming practices can help you avoid uncomfortable situations with your team members. Here’s an anecdote from my early university years when I collaborated on a project with a fellow student. This event took place during a testing and brainstorming session.
My colleague reached out to inform me that he had found a solution to the problem we were tackling. He created a method named Versi(...).
My initial question was about the meaning behind Versi(...). His response was straightforward yet clever: "Ver si funciona" ("Let’s see if it works").
It brought a smile to our faces in that moment. However, the lack of a solid foundation for naming variables or methods was common practice during the first year of university…just think about how often we encountered the variable x in code.
Summary
In summary, poor naming in software can have a cascading impact on various aspects of the development process, from initial coding to long-term maintenance. Good naming practices are essential for creating clean, understandable, and maintainable code. Before construction begins, spell out the programming conventions to be used.
References
- Martin, R. C. (2008). Clean Code: A Handbook of Agile Software Craftsmanship. Prentice Hall.
- McConnell, S. (2004). Code Complete: A Practical Handbook of Software Construction (2nd ed.). Microsoft Press.
- Cornell, C. (2022, March 20). Challenges in Naming Things in Software and Data Engineering. Chris Cornell the Dataist. URL
- Jain, A. (2021, Jan 25). Naming Conventions 101 for developers. URL
- Microsoft Corporation. (2021). .NET Framework Design Guidelines: Naming Guidelines. URL